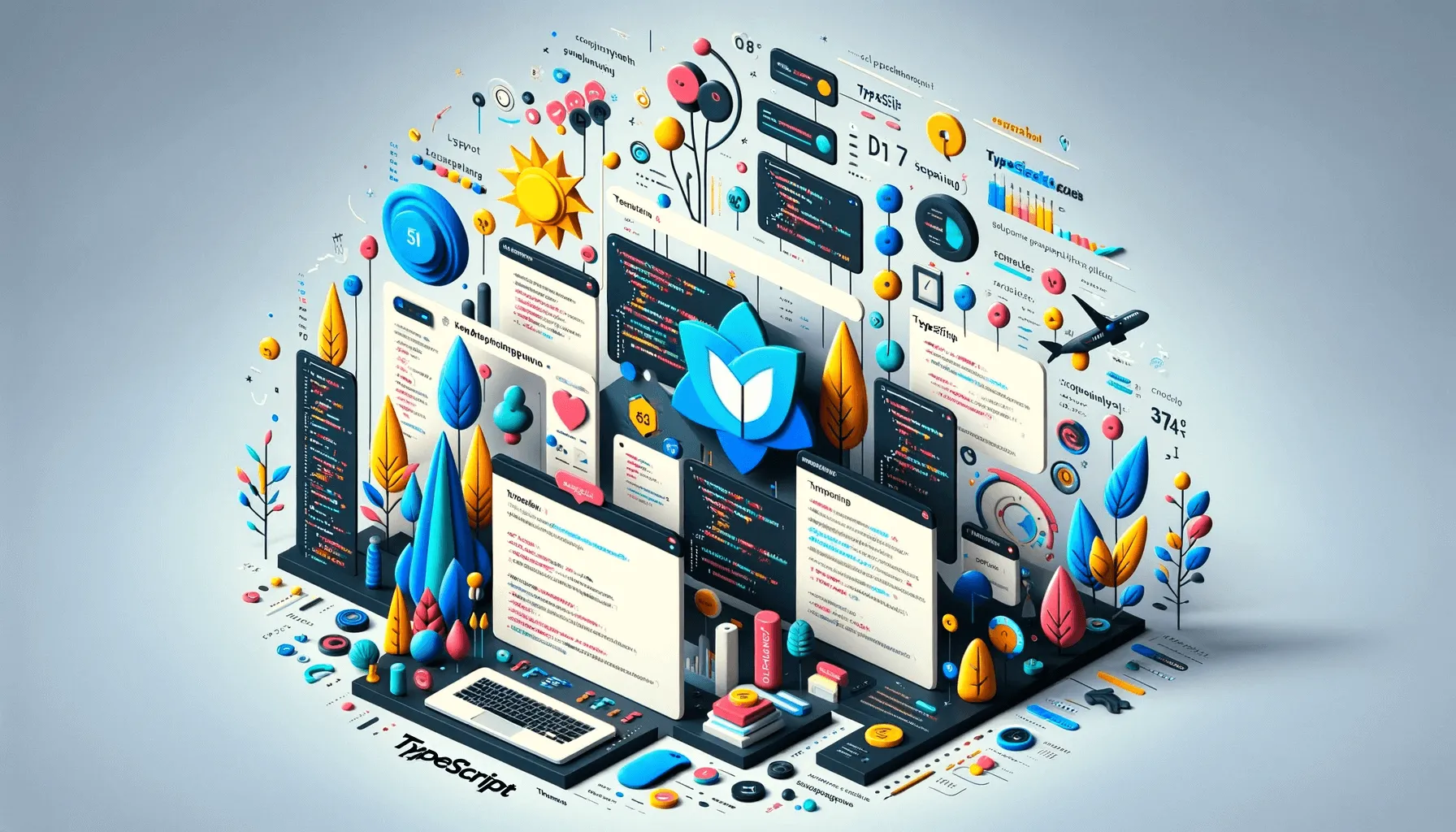
SOLID Principles in TypeScript: Enhancing Software Design and Maintainability
Introduction
In the evolving world of software development, the SOLID principles stand as a beacon, guiding developers towards writing more maintainable, scalable, and efficient code. Originally introduced by Robert C. Martin, these principles are crucial for anyone looking to refine their coding practices, especially in versatile languages like TypeScript. This article delves into each of the SOLID principles, providing practical TypeScript examples to illuminate these concepts clearly.
S: Single Responsibility Principle (SRP)
This principle states that a class should have only one reason to change, meaning it should only have one job or responsibility. By adhering to SRP, software becomes easier to maintain and understand, as each class has a clear purpose and less complexity.
Correct Usage:
// A class with a single responsibility
class User {
private name: string;
constructor(name: string) {
this.name = name;
}
getName(): string {
return this.name;
}
}
// Separate class for database operations
class UserDatabase {
saveUser(user: User) {
// Logic to save the user to a database
}
}
Violation:
// A class with multiple responsibilities
class User {
private name: string;
constructor(name: string) {
this.name = name;
}
getName(): string {
return this.name;
}
saveUser() {
// Logic to save the user to a database
}
}
O: Open/Closed Principle (OCP)
According to this principle, classes should be open for extension but closed for modification. This means that the behavior of a class can be extended without modifying its source code, typically through the use of interfaces or abstract classes. This principle helps in improving the scalability of the software and reduces the risk of breaking existing functionality.
Correct Usage:
abstract class Shape {
abstract calculateArea(): number;
}
class Rectangle extends Shape {
constructor(private width: number, private height: number) {
super();
}
calculateArea(): number {
return this.width * this.height;
}
}
class Circle extends Shape {
constructor(private radius: number) {
super();
}
calculateArea(): number {
return Math.PI * this.radius * this.radius;
}
}
Violation:
class Shape {
constructor(private shapeType: string, private dimensions: number[]) {}
calculateArea(): number {
if (this.shapeType === "rectangle") {
return this.dimensions[0] * this.dimensions[1];
} else if (this.shapeType === "circle") {
return Math.PI * this.dimensions[0] * this.dimensions[0];
}
return 0;
}
}
L: Liskov Substitution Principle (LSP)
This principle states that objects of a superclass should be able to be replaced with objects of a subclass without affecting the correctness of the program. It ensures that a subclass can stand in for its superclass, leading to more robust and reliable software by enforcing consistent behavior across related classes.
Correct Usage:
class Bird {
fly() {
// Flying logic
}
}
class Duck extends Bird {}
function makeBirdFly(bird: Bird) {
bird.fly();
}
const duck = new Duck();
makeBirdFly(duck); // Duck can substitute Bird
Violation:
class Bird {
fly() {
// Flying logic
}
}
class Penguin extends Bird {
fly() {
throw new Error("Cannot fly");
}
}
function makeBirdFly(bird: Bird) {
bird.fly();
}
const penguin = new Penguin();
makeBirdFly(penguin); // Penguin cannot substitute Bird without altering the correctness of the program
I: Interface Segregation Principle (ISP)
The principle advocates for creating small, specific interfaces rather than large, general-purpose ones. By doing so, classes don't have to implement interfaces they don't use, reducing the burden of unnecessary dependencies and making the software more modular and easier to understand.
Correct Usage:
interface Printable {
print(): void;
}
interface Scannable {
scan(): void;
}
class AllInOnePrinter implements Printable, Scannable {
print() { /* printing logic */ }
scan() { /* scanning logic */ }
}
class SimplePrinter implements Printable {
print() { /* printing logic */ }
}
Violation:
interface MultiFunctionDevice {
print(): void;
scan(): void;
}
class SimplePrinter implements MultiFunctionDevice {
print() { /* printing logic */ }
scan() {
throw new Error("Scan function not supported");
}
}
D: Dependency Inversion Principle (DIP)
This principle suggests that high-level modules should not depend on low-level modules, but both should depend on abstractions. Additionally, these abstractions should not depend on details, but details should depend on abstractions. DIP leads to more decoupled and thus easily maintainable and testable code.
Correct Usage:
interface Database {
save(data: string): void;
}
class MongoDatabase implements Database {
save(data: string) { /* MongoDB saving logic */ }
}
class DataProcessor {
private database: Database;
constructor(database: Database) {
this.database = database;
}
processData(data: string) {
this.database.save(data);
}
}
const mongoDB = new MongoDatabase();
const processor = new DataProcessor(mongoDB);
Violation:
class MongoDatabase {
save(data: string) { /* MongoDB saving logic */ }
}
class DataProcessor {
private database: MongoDatabase;
constructor() {
this.database = new MongoDatabase();
}
processData(data: string) {
this.database.save(data);
}
}
const processor = new DataProcessor();
Conclusion
In conclusion, the SOLID principles offer a foundational framework for writing clean, efficient, and maintainable code in TypeScript. Each principle: Single Responsibility
, Open/Closed
, Liskov Substitution
, Interface Segregation
, and Dependency Inversion
, caters to a specific aspect of software design and development. By adhering to these principles, developers can avoid common pitfalls such as tightly coupled code, inflexibility in the face of changing requirements, and difficulty in testing and maintaining the software.
Adopting SOLID principles does require a disciplined approach and a shift in mindset. It involves thinking more about the design of the software from the outset and being mindful of how changes in one part of the system could affect others. However, the long-term benefits such as easier debugging, simpler updates, and more readable code far outweigh the initial effort.
In TypeScript, the application of these principles is particularly effective due to the language's support for strong typing, classes, and interfaces. This support enables clear, explicit, and enforceable coding practices that align well with the SOLID principles. The practical examples provided in this article demonstrate how TypeScript's features can be leveraged to adhere to these principles, ultimately leading to higher quality software.
As the software development landscape continues to evolve, the importance of maintaining and scaling complex systems only increases. In this context, SOLID principles serve not just as guidelines, but as essential tools for any developer aiming to build robust, efficient, and adaptable software. Whether you are a beginner or an experienced developer, integrating these principles into your TypeScript coding practices will undoubtedly yield significant benefits, both for you and for the software you develop.