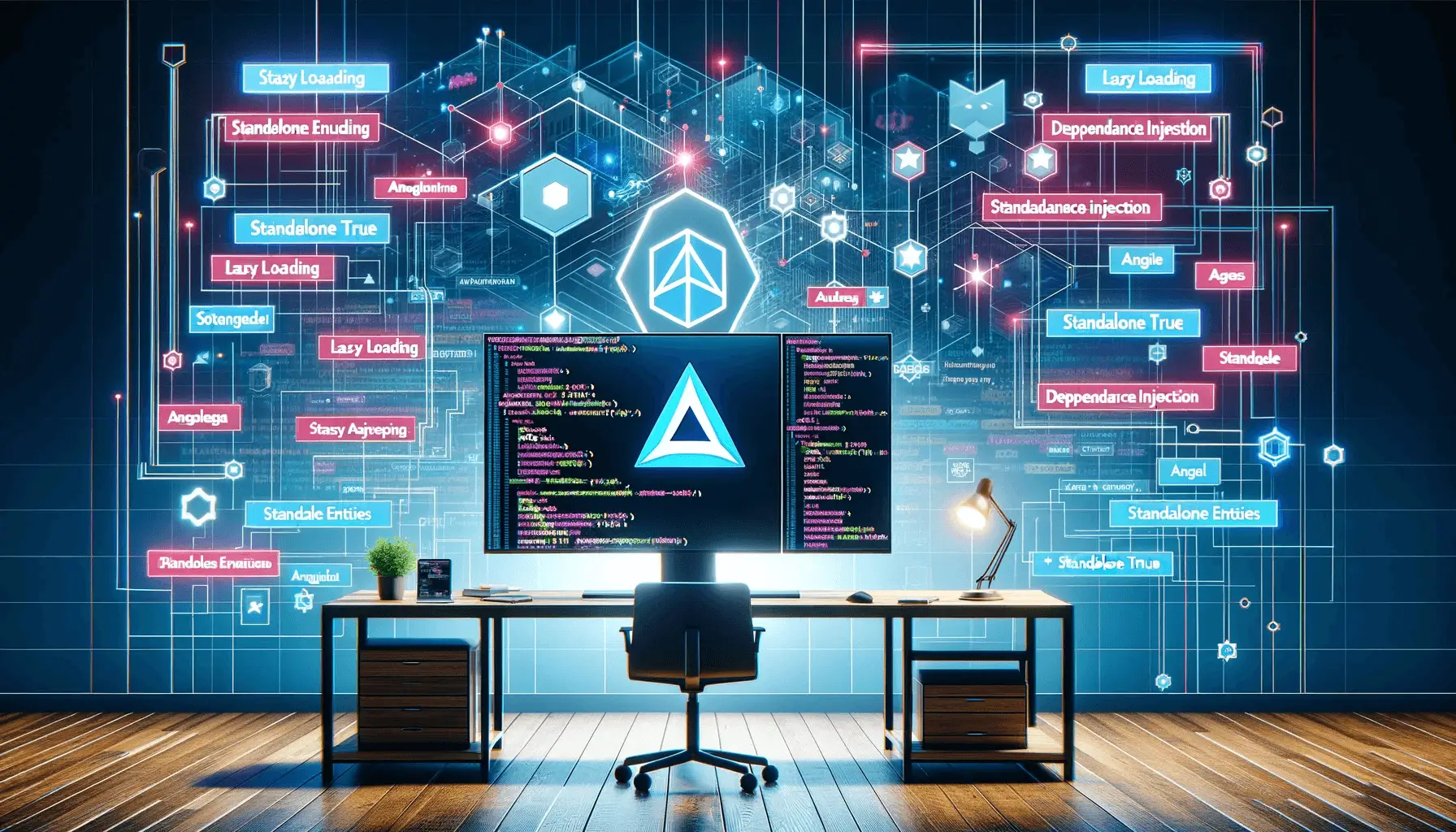
Empowering Angular Development with Standalone Components
Introduction
Angular has always been at the forefront of delivering robust solutions to meet the evolving needs of the modern web. With the introduction of Angular 14, the framework has taken a gigantic leap with the feature of Standalone Components. This revolutionary feature has opened up new avenues for developers to create more modular, reusable, and maintainable applications. Let's delve into the world of Standalone Components and explore how they are changing the Angular landscape.
Understanding Standalone Components Standalone
Components are a new breed of Angular components that are not tied to any specific Angular module. Unlike the traditional approach where components needed to be declared within an Angular module, Standalone Components break free from this requirement.
import { Component } from '@angular/core';
@Component({
standalone: true,
selector: 'app-cart-item',
template: '<p>Item: {{ item.name }}, Price: {{ item.price }}</p>',
})
export default class CartItemComponent {
item = { name: 'Apple', price: 1.25 };
}
In the snippet above, the standalone: true property signifies that CartItemComponent is a Standalone Component.
Creating Standalone Entities
Creating Standalone Components, directives, or pipes is a breeze with Angular. Utilize the --standalone flag in the ng generate component command, and Angular will do the rest.
ng g c cartItem --standalone
This command generates a standalone component named CartItemComponent.
import { CommonModule } from '@angular/common';
@Component({
standalone: true,
selector: 'app-cart-item',
templateUrl: './cart-item.component.html',
styleUrls: ['./cart-item.component.scss'],
imports: [CommonModule],
})
export class CartItemComponent { }
Integrating Standalone Components
You can integrate CartItemComponent within other standalone or module-based components.
import { Component } from '@angular/core';
import { CartItemComponent } from './cart-item/cart-item.component';
@Component({
selector: 'app-cart',
template: '<app-cart-item></app-cart-item>',
imports: [CartItemComponent],
})
export class CartComponent {}
Lazy Loading and Standalone Components
Lazy loading can significantly improve your app’s performance.
const routes: Routes = [
{
path: 'cart-item',
loadChildren: () => import('./path/to/cart-item.component')
}
];
Dependency Injection (DI) in Standalone Components
Managing dependencies is simple and effective within standalone components.
import { Component, inject } from '@angular/core';
import { ItemService } from '../services/item.service';
@Component({
selector: 'app-cart-item',
template: '<p>Item: {{ item.name }}, Price: {{ item.price }}</p>',
providers: [ItemService],
})
export default class CartItemComponent {
item = inject(ItemService).getItem();
}
By specifying providers at the component level, Standalone Components can efficiently manage their dependencies.
Official doc: https://angular.io/guide/standalone-components
Conclusion:
The introduction of Standalone Components in Angular 14 marks a significant stride towards a more modular and manageable application structure. The ease of creation, integration, and the support for lazy loading and dependency injection makes Standalone Components a powerhouse feature for modern-day Angular development. Embracing Standalone Components will undoubtedly lead to cleaner code, faster development cycles, and a noteworthy reduction in the complexity of Angular projects.