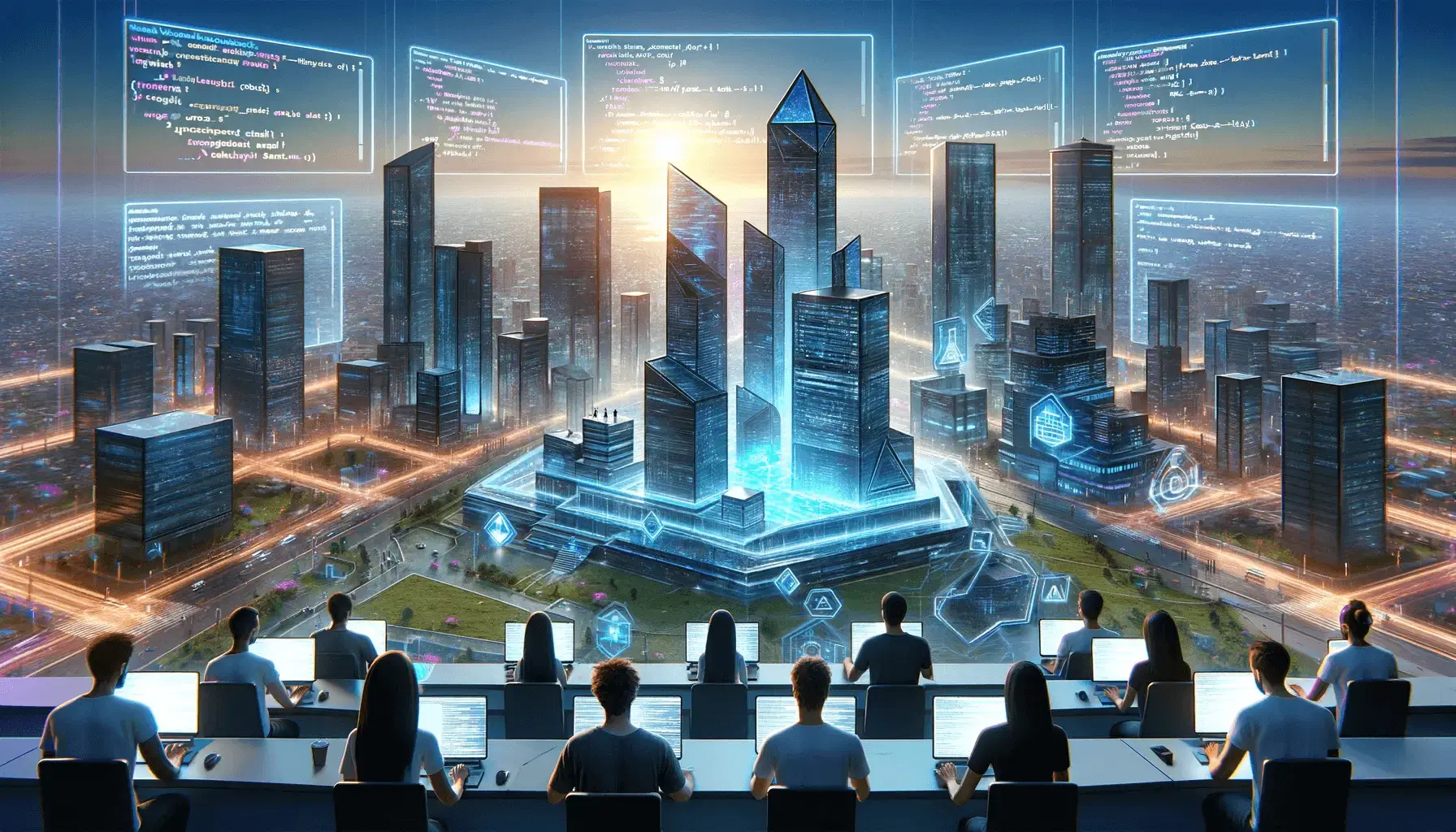
Angular 17 - The Renaissance
Introduction
Angular 17 is a major release of the popular JavaScript framework, and it brings with it a number of new features and improvements. In this article, we will take a look at some of the most notable changes, including the new control flow syntax, the defer block, the SSR hydration improvements, the View Transitions API, and the performance improvements.
Control Flow Syntax
Angular 17 introduced a new control flow syntax, which is currently in preview mode. The new syntax is based on the concept of signals, which are a way to communicate between components and their templates. Signals are similar to events, but they are more specific and can be used to control the rendering of a template.
The new control flow syntax is more declarative and easier to read than the current syntax. It also has a number of performance benefits.
The @-syntax
The new control flow syntax uses the @ character to identify control flow blocks. For example, to render a template only if a certain condition is met, you would use the following syntax:
@if (myCondition) {
<p>{{ conditionText }}</p>
}
You can also do more complex conditions:
@if (product().isChargeable) {
<product-price [product]="product()" />
} @else if (product().isGiftProduct) {
<gift-product-price [product]="product()" />
} @else {
<span>The product is unknown!</span>
}
You can also do loops with a build in trackBy solution:
@for (product of products(); track product.id) {
<span>{{ product.name }}</span>
} @empty {
<span>There were no products in the list.</span>
}
Last but not least the equivalent of the *ngSwitch:
@switch (product().color) {
@case (productColor.red) {
<span>The red one.</span>
}
@case (productColor.blue) {
<span>The blue one.</span>
}
@default {
<span>The let us surprise you color.</span>
}
}
Defer Block
The defer block is a template construct that is used to define content that will be loaded lazily. When the defer block is encountered, Angular will compile the content inside the block into a separate chunk. This chunk will not be loaded until the defer block is triggered.
There are two ways to trigger a defer block:
- When: The defer block will be triggered when a specific condition is met. For example, you could trigger a defer block when a user scrolls to a certain position on the page.
- On: The defer block will be triggered when a specific event occurs. For example, you could trigger a defer block when a user clicks on a button.
There are a number of benefits to using the defer block:
Improved performance: Lazy loading components, directives, and pipes can significantly improve the performance of your Angular applications. This is because the browser only needs to load the resources that are actually being used.
- Reduced bundle size: Lazy loading can also reduce the bundle size of your Angular applications. This is because the browser only needs to download the resources that are actually being used.
- Improved user experience: Lazy loading can also improve the user experience of your Angular applications. This is because users will not have to wait for all of the resources to be loaded before they can start using the application.
Here are a few examples of how to use the defer block:
<!-- On viewport -->
@defer (on viewport(trigger)) {
<product/>
}
<!-- If the condition has been met -->
<button (click)="showContent.set(true)">Show product</button>
@defer (when showContent()) {
<product/>
}
SSR hydration improvements
Overall, the hydration improvements in Angular 17 make SSR applications faster, more responsive, and more user-friendly.
- Non-destructive hydration: This is the biggest improvement, and it means that Angular can now reuse the DOM that was rendered on the server, without having to destroy and re-render it entirely. This can significantly improve the performance of SSR applications.
- Improved hydration of dynamic content: Angular can now better hydrate dynamic content, such as content that is generated using templates. This can help to improve the user experience of SSR applications.
- Reduced flickering: Angular has also made a number of improvements to reduce flickering when hydrating SSR applications.
View Transitions API
Angular 17 introduces a new and exciting feature: the View Transitions API. This API allows you to create smooth and engaging animations when transitioning between different views in your application.
The View Transitions API works by creating a pseudo element tree. This is a temporary copy of the DOM that is used to represent the state of the DOM before and after the transition. Angular then animates the pseudo element tree to create the transition effect.
Benefits of using the View Transitions API, there are a number of benefits to using the View Transitions API:
- It is easy to use.
- It is very powerful and allows you to create complex animations.
- It is performant.
- It is compatible with browsers (Browser compatibility).
How to enable it by using the new provider syntax:
import { provideRouter, withViewTransitions } from '@angular/router';
import { APP_ROUTES } from 'src/app/app.routes';
bootstrapApplication(AppComponent, {
providers: [
provideRouter(
APP_ROUTES, // Your route configurations
withViewTransitions() // View Transitions
),
],
})
You can also use the View Transitions API to create custom animations that match your application's unique style. For example, you could create an animation that causes a new view to appear from behind an existing view, or an animation that causes a view to shrink and disappear into thin air.
The View Transitions API is a powerful new feature in Angular 17 that can help you to create smooth and engaging animations when transitioning between different views in your application. If you are not already using the View Transitions API, I encourage you to give it a try.
The Performance of Angular 17
Angular 17 is one of the fastest JavaScript frameworks available, and it is significantly faster than Angular 16. This is due to a number of improvements that have been made to the Angular compiler and the Ivy rendering engine.
Here are some of the performance improvements in Angular 17:
- Faster code generation: The Angular compiler has been optimized to generate faster code. This can lead to a significant performance improvement for applications that have a large amount of code.
- More efficient rendering: The Ivy rendering engine has been optimized to be more efficient. This can lead to a significant performance improvement for applications that have a lot of dynamic content.
- Reduced bundle size: Angular 17 has a smaller bundle size than Angular 16. This can lead to a faster load time for your applications.
The following benchmark results show how Angular 17 performs compared to other popular JavaScript frameworks:
Framework | Time to render 10000 items (ms) |
Angular 17 | 13.2 |
React 18 | 18.5 |
Vue 3 | 20.1 |
As you can see, Angular 17 is significantly faster than React and Vue.
Here are some tips for improving the performance of your Angular 17 applications:
- Use the View Transitions API to create smooth and engaging animations when transitioning between different views in your application.
- Use lazy loading to load modules and components only when they are needed.
- Use the Angular CLI to bundle your application for production.
- Use a CDN to deliver your application's assets.
By following these tips, you can create Angular 17 applications that are fast and responsive.
Reference: https://krausest.github.io/js-framework-benchmark/current.html
Conclusion
Angular 17 is a major release of the popular JavaScript framework, and it brings with it a number of new features and improvements. The new control flow syntax, the defer block, the SSR hydration improvements, the View Transitions API, and the performance improvements all make Angular 17 a more powerful and user-friendly framework.
If you are developing Angular applications, I encourage you to upgrade to Angular 17 today. You will be glad you did.